@markdown
# AJAX 비동기 처리 방식 사용하기
____
## AJAX(<u>A</u>synchronous <u>J</u>avascript <u>A</u>nd <u>X</u>ml)
- 비동기 방식의 자바스크립트 XML
- 웹 페이지 일부 영역 부분만 비동기 처리 방식으로 화면이 바뀔 수 있게 해준다.
- 백그라운드 작업을 통해서 일부분의 화면만 새로고침 없이 요청하겠다는 것
- 동기 방식인 URL 대신 서버에 요청해 비동기 통신을 통해 응답을 받는다
- 보통 XML, JSON 데이터가 날라온다.
- 사용 예) 구글 지도, 페이스북 타임라인 등
## AJAX 동작 구조
____
- AJAX 엔진에서 비동기 처리 부분을 가로채 백그라운드에서 실행하는 방식

## XMLHttpRequest
____
- 백그라운드로 `Http` 프로토콜을 이용한 서버와의 요청 / 응답을 처리하는 자바스크립트 객체
- 서버와 통신시 비동기적으로 작업
- 대부분의 웹 브라우저에서 지원한다.
1. 클라이언트 `event` 발생
2. `XMLHttpRequest` 생성
3. `post` 방식 비동기 요청
4. `Web Container`에서는 유효한 `Servlet`인지 확인
5. DB 체크 후 결과 응답
6. `XML` 또는 `JSON` 데이터로 `XMLHttpRequest`에 응답
7. 클라이언트 이벤트 처리
### open("Http method", "URL", syn/asyn)
- 요청 초기화 작업
- `get` / `post` 지정
- 요청할 jsp url 지정
- 동기 / 비동기 설정(true : 비동기 방식)
### send(param)
- 실제 비동기 요청하는 함수
- get방식은 url에 데이터가 붙을 것이기 때문에 `null` 입력
- post 방식은 param을 통해 데이터 전달
## AJAX javascript 코드
____
<pre><code class="html" style="font-size:14px">Hello01.jsp(비동기 호출할 jsp)
<script>
// XMLHttpRequest 객체 저장할 변수 선언
var httpRequest = null;
function requestMsg(){
//AJAX 통신 준비
//1. XMLHttpRequest 객체 생성
// window 버전이 낮을 경우 대비한 객체 생성
if(window.XMLHttpRequest){
httpRequest = new XMLHttpRequest();
} else if(window.ActiveXObject){
httpRequest = new ActiveXObject("Microsoft.XMLHTTP");
} else {
httpRequest = null;
}
//alert(httpRequest);
// 2.콜백 함수 설정
httpRequest.onreadystatechange = responseMsg;
// 3. 서버에 비동기 요청
httpRequest.open("GET", "hello.jsp", true);
httpRequest.send(null);
}
// 4. 서버 응답의 완료를 처리할 함수
function responseMsg(){
if(httpRequest.readyState == 4){
if(httpRequest.status == 200){
alert(httpRequest.responseText);
var div = document.getElementById("msgView");
//httpRequest.responseText를 통해 jsp 데이터를 가져온다.
div.innerHTML = httpRequest.responseText;
}
}
}
</script>
</code></pre>
<br/>
### httpRequest 출력
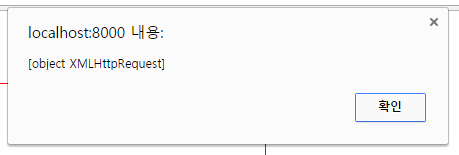
### Chrome 개발자모드 Network 탭에서 비동기 호출 확인
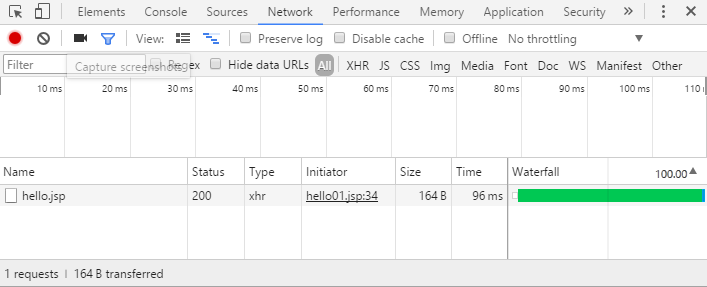
- Ajax는 백그라운드 방식이기 때문에 Network로 반드시 확인해야한다.
## HTML 코드
____
<pre><code class="html" style="font-size:14px"><body>
<h2>서버에서 받은 메시지</h2>
<div id="msgView">
</div>
<input type="button" value="서버에 자료 전송" onclick="requestMsg()"/>
</body>
</html>
</code></pre>
## 비동기 호출 hello.jsp
____
<pre><code class="html" style="font-size:14px"><%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<h2>Hello World</h2>
</code></pre>
### onreadystatechange
- 서버에서 응답이 도착했을때 호출될 콜백함수 지정
- `httpRequest.onreadystatechange = callbackMethod()`
- 요청에 대한 서버 응답이 바뀔때마다 `httpRequest.readyState` 값이 바뀐다.
### readyState 통한 비동기 처리 확인.
- 0 : open() 호출전
- 1 : open() 호출, send() 호출전
- 2 : send() 호출, 서버 응답전
- 3 : 서버응답, header 전송, body 전송
- 4 : 클라이언트에서 body 전송완료(응답완료)
```
if(httpRequest.readyState == 4)
if(httpRequest.status == 200)
```
- 서버에 대한 응답이 완료되야 status를 확인 후 클라이언트에 출력해줄 수 있다.(호출 순서 바뀌면 안됨)
## Servlet 사용한 Ajax 비동기 호출
____
- Java Resources - src 경로에 servlet 파일 생성
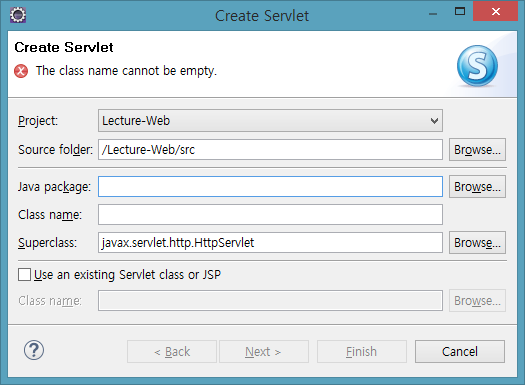
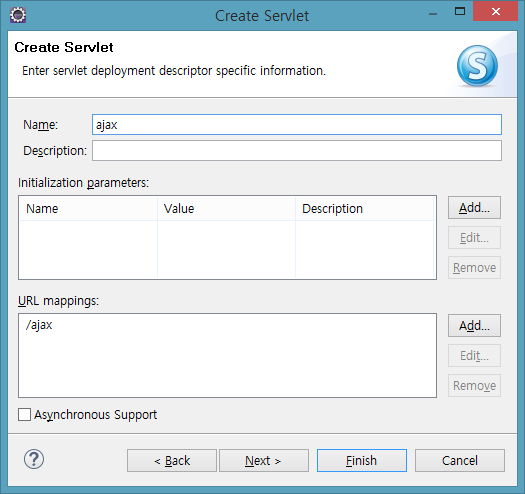
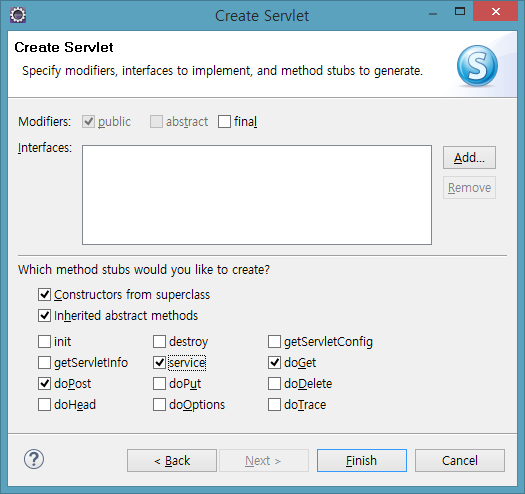
### Servlet 처리 코드
- 비동기 요청하는 `httpRequest.open` url 파라미터에 `URL mappings`에서 매핑해준 이름을 호출한다.
```
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.println("<h1>Hello Ajax</h1>");
out.flush();
out.close();
}
```
### Post 비동기 호출 인코딩 설정
- Post 방식은 `form`의 내용을 보내는 것이기 때문에 `form` 태그를 사용하지 않고 전송하기 위해서 url 인코딩을 해준다.
```
httpRequest.open("POST", url, true);
httpRequest.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
httpRequest.send(args);
```
## jQuery ajax 라이브러리 사용
____
- jQuery에서 제공되는 ajax 함수 호출을 통해 간편하게 사용할 수 있다.
<pre><code class="html" style="font-size:14px"><script>$(document).ready(function() {
jQuery.ajax({
type:"GET",
url:"/test",
dataType:"JSON", // json, param 등 다양하게 사용 가능(옵션)
success : function(data) {
// 통신이 성공적으로 이루어졌을 때 실행
},
complete : function(data) {
// 통신 실패 or 완료가 되었을 때 호출
},
error : function(xhr, status, error) {
alert("에러발생");
}
});
});
</script>
</code></pre>
'웹 프로그래밍' 카테고리의 다른 글
Spring - 핵심 개념(IoC, DI) (0) | 2017.07.05 |
---|---|
Spring - Maven 설치 및 Eclipse 환경설정 하기 (0) | 2017.07.04 |
Ajax 사용한 Daum 검색 API 사용하기 (0) | 2017.06.21 |
JSP, jQuery로 간단한 텍스트 편집기 만들기 (1) | 2017.06.13 |
Tomcat 웹 서버 설치하기 (0) | 2017.05.30 |